On this page
article
3.JavaScript
使网页具有交互性,例如响应用户点击,给用户提供更好的体验
1.JavaScript发展史
- JavaScript(简称JS):是一种轻量级客户端脚本语言,通常被嵌入HTML页面,在浏览器上执行。
- JavaScript的主要用途:
- 使网页具有交互性,例如响应客户点击,给用户提供更好的体验。
- 处理表单,检测用户输入,并及时反馈提醒。
- 浏览器与服务端之间数据通信,主要通过Ajax异步传输。
- 在网页中添加标签,添加样式,改变标签的属性等。对Dom节定进行操作
2.HelloWorld
<!DOCTYPE html>
<html lang="en" xmlns="http://www.w3.org/1999/html" xmlns="http://www.w3.org/1999/html">
<head>
<meta charset="UTF-8">
<title>首页</title>
</head>
<body>
<p>Hello Js</p>
<script>
var name = 'Hello JavaScript';
alert(name);
</script>
</body>
</html>
3.事件
事件 | 描述 |
---|---|
onclick |
点击 HTML 元素时触发 |
onmouseover |
鼠标移动到元素上时触发 |
onkeydown |
按下键盘按键时触发 |
onchange |
HTML 元素发生变化时触发 |
onmouseout |
鼠标移开 HTML 元素时触发 |
onload |
页面加载完成时触发 |
<!DOCTYPE html>
<html lang="en" xmlns="http://www.w3.org/1999/html" xmlns="http://www.w3.org/1999/html">
<head>
<meta charset="UTF-8">
<title>首页</title>
</head>
<body>
<button onclick="alert('点击时触发')">onclick</button>
<button onmouseenter="alert('鼠标移动上来时触发')">onmouseenter</button>
<button onmouseout="alert('鼠标移开时触发')">onmouseout</button>
<br>
输入你的名字:<input type="text" id="fName" onchange="myfunction()" />
<script>
function myfunction(){
var inputName = document.getElementById("fName")
inputName.value = inputName.value.toUpperCase() /*转成大写*/
}
/*页面加载完毕时触发*/
window.onload = f;
function f(){
alert('页面加载完毕')
}
</script>
</body>
</html>

第一个点击触发,第二个鼠标移动上去触发,第三个鼠标移出时触发。输入框焦点改变时触发
4.选择器
- JS选择器主要用来获取HTHL页面中的元素,将页面中的元素保存到一个对象中,然后就可以对这些对象的属性值进行相应操作,以实现一些动态效果,以达到页面的生动,易用。
- 需要注意的一点是操作的一定是对象,直接将元素当做对象使用是不行的。
- 常见选择器:标签选择器、类选择器、ID选择器
<html>
<head>
<meta http-equiv="Content-Type" content="text/html;charset=UTF-8">
<title>Document</title>
</head>
<body>
<button id="btn">点我</button>
<div id="main">
<p>p1</p>
<p>
<a style="color: dodgerblue">我是p2下的a标签</a>
</p>
<p>p3</p>
</div>
<script>
var btnid = document.getElementById('btn') /*获取id为btn的元素*/
btnid.onclick = function (){ //为btn按钮绑定一个点击事件
alert('点我干神魔')
}
var x = document.getElementById('main')
var y = document.getElementsByTagName('p') /*返回一个数组,通过下标获取内容*/
console.log(x,y)
document.write('div中第二段文本是'+y[1].innerHTML) //向当前文档写入内容
</script>
</body>
</html>
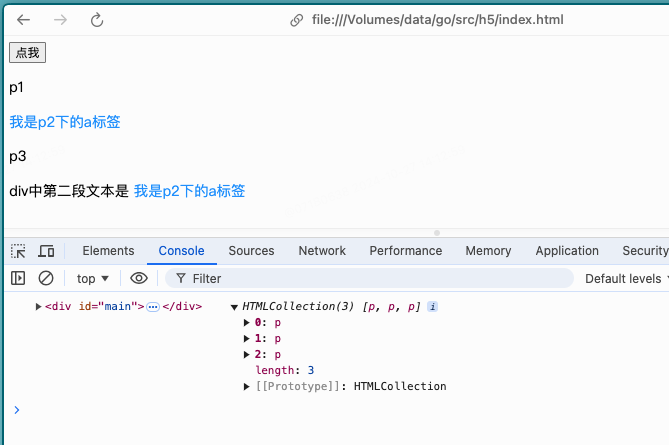
5.JS操作html
- 插入内容
document.write("<p>这是JS写入的段落</p>");
//向文档写入HTML内容x = document.getElementById('demo');
//获取id为demo的元素x.innerHTML="<a>Hello"</a>"
//向元素插入HTML内容 - 改变标签属性
document.getElementById("image").src="b.jpg"
//修改img标签src属性值 - 改变标签样式
x = document.getElementById("p")
//获取id为p的元素x.style.color="blue"
//字体颜色
<html>
<head>
<meta http-equiv="Content-Type" content="text/html;charset=UTF-8">
<title>Document</title>
</head>
<body>
<input id="in1"/>
<p>Hello</p>
<script>
a = document.getElementById('in1');
a.type = "button";
a.value = "按钮";
a.onclick = function (){ /*绑定点击事件*/
b = document.getElementsByTagName('p'); /*获取p标签对象*/
b[0].style.color = "red" /*修改第一个p标签颜色*/
b.item(0).innerHTML = b[0].innerHTML+"点击按钮修改了内容" /*在原来内容的基础上,增加内容*/
}
</script>
</body>
</html>
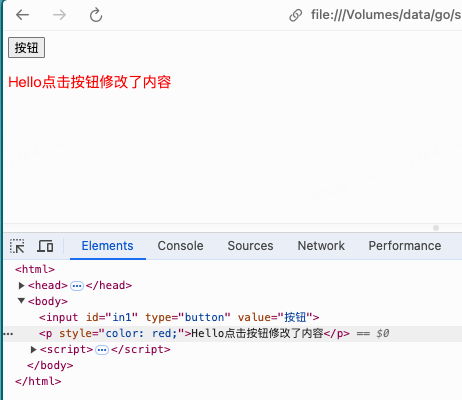
5.1字符串
<html>
<head>
<meta http-equiv="Content-Type" content="text/html;charset=UTF-8">
<title>Document</title>
</head>
<body>
<script>
var s = 'hello world'
//获取字符串长度
console.log("字符串长度:",s.length)
//根据下标获取值
console.log("第五个字符是:",s[4])
//替换字符串
console.log(s.replace('h',"H"))
//字符串转数组
console.log(s.split(' '))
//数组转字符串
var sarr = s.split(' ')
console.log(sarr.join(','))
//找到返回匹配的字符,否则返回null
console.log(s.match('w'))
//字符串拼接
console.log(s + "aaa")
</script>
</body>
</html>
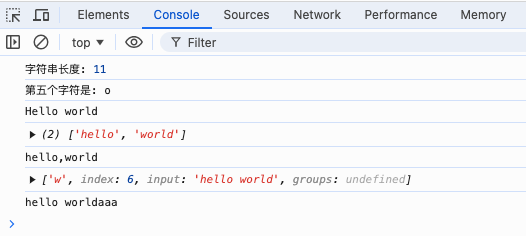
5.2数组
<html>
<head>
<meta http-equiv="Content-Type" content="text/html;charset=UTF-8">
<title>Document</title>
</head>
<body>
<script>
//数组定义
var arr = new Array()
var arr1 = []
//添加元素
arr[0] = "衣服"
arr[1] = "鞋子"
arr[2] = "帽子"
arr.push("裤子")
console.log(arr)
//通过索引查找元素
console.log(arr[1])
//数组长度
console.log(arr.length)
//数组遍历
for (var i=0;i<arr.length;i++){
console.log(arr[i])
}
//或者
arr.forEach(function (element,index){
console.log(element,index)
})
//删除元素
console.log(arr.slice(0,arr.length - 1))
</script>
</body>
</html>
5.3对象
- 对象是一个具有映射关系的数据结构,对于存储有一定的关系的元素。
- 格式:
d = {'key1':value1,'key2':value2,'key3':value3}
- 注意:对象通过
key
来访问value
,所以key
不允许在同一个字典中重复。
<html>
<head>
<meta http-equiv="Content-Type" content="text/html;charset=UTF-8">
<title>Document</title>
</head>
<body>
<script>
//对象的定义
var user = {
name :'张三',
sex :'男',
age:'18'
}
//或者
var user1 = new Object
console.log(user)
//通过属性名查询值
console.log(user.name)
//或者
console.log(user['name'])
//增加或修改
user.height = '180cm'
console.log(user)
user['height'] = '175cm'
console.log(user)
</script>
</body>
</html>
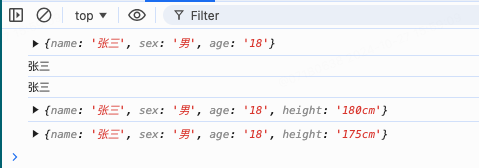
6.操作符、流程控制、函数
6.1操作符
- 一个特定的符号,与其他数据类型连接起来组成一个表达式,常用于条件判断,根据表达书返回Ture或False采取动作
<html>
<head>
<meta http-equiv="Content-Type" content="text/html;charset=UTF-8">
<title>Document</title>
</head>
<body>
<script>
//比较操作符,在js中 == 只判断最终的值是否相等;=== 会判断类型
console.log('1' == 1) //true
console.log('1' === 1) //false
//算数操作符
console.log(1+2)
var num = 0
num ++
console.log(num)
//逻辑操作符
console.log(num > 0 && num > -1)
//赋值操作符
console.log(num += 1)
</script>
</body>
</html>
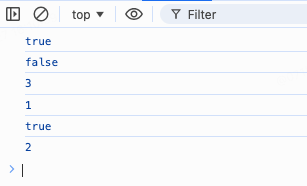
6.2流程控制
- if条件判断:判定给定的条件是否满足(True或False), 根据判断的结果决定执行的语句。
语法:
if (表达式) {
<代码块>
} else if (表达式) {
<代码块>
} else {
<代码块>
}
<!--实现一个开关灯-->
<html>
<head>
<meta http-equiv="Content-Type" content="text/html;charset=UTF-8">
<title>Document</title>
<style>
#laodeng img {
height: 150px;
}
</style>
</head>
<body>
<div id="laodeng">
<img id="laodeng-img" src="./img/close.png">
<input id="laodeng-switch" type="button" onclick="changeImage('on')" value="开启"/>
</div>
<script>
myswitch = document.getElementById('laodeng-switch')
myimg = document.getElementById('laodeng-img');
function changeImage(status){
if (status == 'on') {
myswitch.value = '关闭'
myswitch.setAttribute('onclick', "changeImage('off')");
myimg.src="./img/open.png"
} else {
myswitch.value = '开启'
myswitch.setAttribute('onclick', "changeImage('on')");
myimg.src="./img/close.png"
}
}
/*
setAttribute 是一个 DOM 方法,用于设置指定元素的属性值。这个方法非常灵活,可以用于修改标准属性、添加自定义数据属性等。以下是 setAttribute 方法的详细说明和示例。
语法:element.setAttribute(attributeName, value);
element: 要操作的 DOM 元素。
attributeName: 要设置的属性名(必须是字符串)。
value: 要赋给该属性的值(可以是字符串或其他类型)。
*/
</script>
</body>
</html>
- for循环:一般用于遍历数据类型的元素进行处理,例如字符串、数组、对象。
<html>
<head>
<meta http-equiv="Content-Type" content="text/html;charset=UTF-8">
<title>Document</title>
</head>
<body>
<script>
var arr = ["debian","centos","ubuntu","redhat"]
//遍历数组方式1
console.log("遍历数组方式1:")
for (i in arr){
console.log(arr[i])
}
//遍历数组方式2
console.log("遍历数组方式2:")
arr.forEach(function (k){
console.log(k)
})
//遍历数组方式3
console.log("遍历数组方式3")
for (var i=0;i<arr.length;i++){
console.log(arr[i])
}
//遍历对象方式1
var user = {
name:"张飞",
age:18,
sex:"男"
}
console.log("遍历对象方式1")
for (k in user){
console.log(user[k])
}
console.log("遍历对象方式2")
//遍历值
Object.values(user).forEach(function (k){
console.log(k)
})
//遍历键
console.log("遍历对象方式3")
Object.keys(user).forEach(function (k){
console.log(k)
})
</script>
</body>
</html>
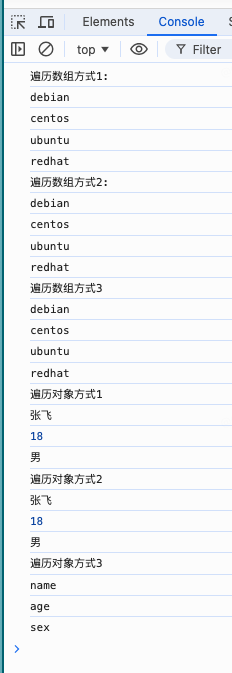
6.3函数
- 函数:是指一段可以直接被另一段程序或代码引用的程序或代码。 在编写代码时,常将一些常用的功能模块编写成函数,放在函数库中供公共使用,可减少重复编写程序段和简化代码结构。
- 普通函数
<html>
<head>
<meta http-equiv="Content-Type" content="text/html;charset=UTF-8">
<title>Document</title>
</head>
<body>
<button type="button" onClick="hello()">你好</button>
<button type="button" onClick="myFunc('张三', '30')">点我</button>
<script>
//定义方法,无参
function hello(){
alert('你不好世界')
}
//定义方法,接收参数
function myFunc(name,age){
alert('欢迎'+age+'的'+name)
}
</script>
</body>
</html>
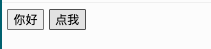
- 匿名函数与箭头函数
<html>
<head>
<meta http-equiv="Content-Type" content="text/html;charset=UTF-8">
<title>Document</title>
</head>
<body>
<script>
//普通函数
function sum1(x,y){
return x+y
}
//匿名函数
sum2 = function (x,y){
return x-y
}
//箭头函数
sum3 = (x,y) => {
return x*y
}
console.log(sum1(1,1),sum2(2,1),sum3(9,9))
</script>
</body>
</html>
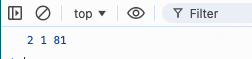
7.window对象
- 在JavaScript中,一个浏览器窗口就是一个window对象。
- 一个窗口就是一个window对象,这个窗口里面的HTML文档就是一个document对象,document对象是window对象的子对象。
- location也是windows的子对象,用于操作URL地址
<html>
<head>
<meta http-equiv="Content-Type" content="text/html;charset=UTF-8">
<title>Document</title>
</head>
<body>
<button type="button" onClick="location.reload()">刷新当前页面</button>
<button type="button" onClick="location.href=location.href">重新请求当前页面</button>
<button type="button" onClick="location.href='https://cakepanit.com'">请求别的页面</button>
</body>
</html>

8.jQuery库介绍
- jQuery:是一个JavaScript的库,极大的简化了JavaScript编程,例如JS原生代码几十行实现的功能,JQuery可能一两行代码就可以实现了,因此得到前端程序员广泛应用。
- 发布至今已经有三个大版本
- 1.x:常用版
- 2.x/3.x:除非特殊要求,一般用的很少
8.1基本使用
- cdn导入方式
<html>
<head>
<meta http-equiv="Content-Type" content="text/html;charset=UTF-8">
<title>Document</title>
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/jquery/1.9.1/jquery.min.js"></script>
</head>
<body>
<script>
// jQuery代码
</script>
</body>
</html>
-
基础语法是:
$(selector).action()
$
代表jQuery本身(selector)
:选择器、查找HTML
元素action()
:对元素进行操作
-
代码示例:按钮实现
<html>
<head>
<meta http-equiv="Content-Type" content="text/html;charset=UTF-8">
<title>Document</title>
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/jquery/1.9.1/jquery.min.js"></script>
</head>
<body>
<button id="btn1">点我1</button>
<button id="btn2">点我2</button>
<script>
// js实现
var x = document.getElementById('btn1')
x.onclick = function (){
alert("你好我是js")
}
//jQuery实现
$('#btn2').click(function (){
alert("你好我是jQuery")
})
</script>
</body>
</html>
8.2选择器
- 选择器介绍
名称 | 语法 | 示例 |
---|---|---|
标签选择器 | element | $("h1") 选择所有的h1的元素 |
类选择器 | .class | $(".title") 选择所有class为title的元素 |
ID选择器 | #id | $("#title") 选取id为title的元素 |
并集选择器 | selector1,selector2,… | $("div,p,.title") 选取所有div、p和拥有class为title的元素 |
属性选择器 | [attr=value] | ("[href='#']") 选取href值等于#的元素 |
- 代码示例
<html>
<head>
<meta http-equiv="Content-Type" content="text/html;charset=UTF-8">
<title>Document</title>
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/jquery/1.9.1/jquery.min.js"></script>
</head>
<body>
<h1>在h5中如何使用jQuery</h1>
<button class="btn">点我1</button>
<button id="btn">点我2</button>
<script>
//标签选择器
$('h1').click(function() {
alert("你好,标签")
})
//类选择器
$('.btn').click(function() {
alert("你好,类")
})
//id选择器
$('#btn').click(function() {
alert("你好,id")
})
</script>
</body>
</html>
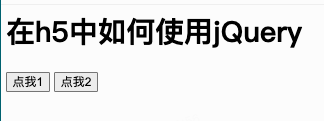
8.3操作html
- 隐藏和显示元素
hide()
:隐藏某个元素show()
:显示某个元素toggle()
:hide()
和show()
方法之间切换
<html>
<head>
<meta http-equiv="Content-Type" content="text/html;charset=UTF-8">
<title>Document</title>
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/jquery/1.9.1/jquery.min.js"></script>
</head>
<body>
<p id="demo">这是一句话</p>
<button id="hide">隐藏</button>
<button id="show">显示</button>
<button id="toggle">切换</button>
<script>
//隐藏是通过css属性绑定实现的
$('#hide').click(function (){
$('#demo').hide()
})
$('#show').click(function (){
$('#demo').show()
})
$('#toggle').click(function (){
$('#demo').toggle()
})
</script>
</body>
</html>
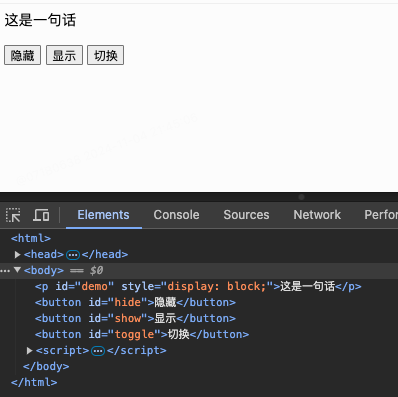
- 获取与设置内容
text()
:设置或返回所选元素的文本内容html()
:设置或返回所选元素的HTML内容val()
:设置或返回表单字段的值
<html>
<head>
<meta http-equiv="Content-Type" content="text/html;charset=UTF-8">
<title>Document</title>
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/jquery/1.9.1/jquery.min.js"></script>
</head>
<body>
<p id="demo">这是一句<b>话</b></p>
<button id="getTxt">获取文本</button>
<button id="getHtml">获取Html</button>
<input type="text" id="input">
<p id="sedTxt"></p>
<p id="sedHtml"></p>
<script>
$('#getTxt').click(function (){
myText = $('#demo').text();
console.log(myText)
$('#sedTxt').text(myText)
//填充文本框
$('#input').val(myText)
})
$('#getHtml').click(function (){
myHtml = $('#demo').html();
console.log(myHtml)
$('#sedHtml').html(myHtml)
//填充文本框
$('#input').val(myHtml)
})
</script>
</body>
</html>
- 设置CSS样式
css()
:设置或返回样式属性addClass()
:向被选中元素添加一个或多个类样式removeClass()
:从被选中元素中删除一个或多个样式toggleClass()
:对被选中元素进行添加/删除类样式的却换动作
<html>
<head>
<meta http-equiv="Content-Type" content="text/html;charset=UTF-8">
<title>Document</title>
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/jquery/1.9.1/jquery.min.js"></script>
<style>
.css1 {
color: brown;
font-size: 20px;
background-color: cornflowerblue;
}
.css2 {
color: cornflowerblue;
font-size: 20px;
background-color: brown;
}
</style>
</head>
<body>
<p id="demo">这是一句话</p>
<button id="sedCss">设置为样式0</button>
<button id="sedCss1">设置为样式1</button>
<button id="sedCss2">设置为样式2</button>
<script>
$('#sedCss').click(function (){
$('#demo').removeAttr("class")
$('#demo').css("color","darkgreen")
})
$('#sedCss1').click(function (){
$('#demo').removeAttr("style class") //删除全部的样式属性和类
$('#demo').addClass("css1") //添加类
})
$('#sedCss2').click(function (){
$('#demo').removeAttr("style class")
$('#demo').addClass("css2")
})
</script>
</body>
</html>
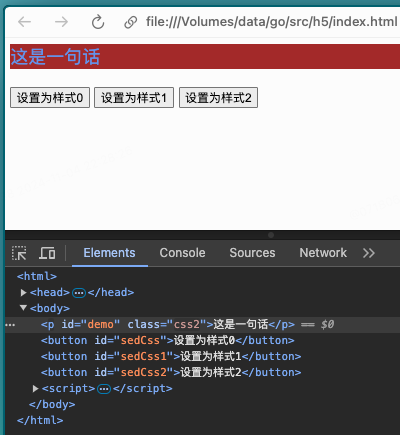
9.Ajax前后端数据交互
- 浏览器访问一个网站的页面时,Web服务器处理完后会以消息体的方式返回给浏览器,浏览器会自动解析HTML内容,如果局部有数据需要更新,需要刷新浏览器重新发起页面请求,获取最新数据,如果每次都是通过这个刷新来解决这个问题,势必会给服务器造成负载,页面加载缓慢。
- Ajax(Asynchronous JavaScript And XML,异步JavaScript和XML),Ajax是一种在无需重新加载整个网页的情况下,能够更新部分网页的技术,例如在你不断刷新页面的情况下查询数据,登录验证等。
- 无刷新的好处:
- 减少带宽,服务器负载
- 提高用户体验
9.1基本使用
- jQuery Ajax主要使用
$.ajax()
方法实现,用于向服务器发送HTTP请求。 - 语法:
$.ajax([settings])
; - settings是
$.ajax()
方法的参数列表,用于配置Ajax请求的键值对集合,参数主要如以下
参数 | 类型 | 描述 |
---|---|---|
url | string | 发送请求地址,默认为当前页面 |
type | string | 请求方式,默认为get |
data | object、array、string | 发送到服务器的数据 |
dataType | string | 预期服务器返回的数据类型,包括Json、XML、Text、HTML等 |
contentType | string | 发送信息到服务器时内容编码类型,默认是application/x-www-form-urlencoded |
timeout | number | 设置请求超时时间 |
headers | object | 设置请求头信息 |
async | Boolean | 默认为true,所有请求均为异步,设置false发送同步请求 |
//请求bing开放壁纸接口。展示到页面上
<html>
<head>
<meta http-equiv="Content-Type" content="text/html;charset=UTF-8">
<title>Document</title>
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/jquery/1.9.1/jquery.min.js"></script>
<style>
.d0 {
/* 要使用flex布局,那么父级元素属性中 必须有display: flex; */
display: flex;
flex-direction: row-reverse; /*逆排序*/
justify-content: center; /*x轴居中*/
flex-wrap: wrap;
}
.d1{
height: 270px;
width: 480px;
background-color: darkcyan;
background-size: 100%;
margin-right: 10px; /*右间距*/
margin-top: 10px;
color:dodgerblue;
text-align: center;
}
</style>
</head>
<body>
<div class="d0">
</div>
<script>
$.ajax({
url:'https://raw.onmicrosoft.cn/Bing-Wallpaper-Action/main/data/zh-CN_update.json',
type:'get',
success: function (result) {
data = JSON.parse(result) //如果响应头中Content-Type: application/json 那么不需要写这一行
for(k in data.images) {
$('.d0').append('<div class="d1" style="background-image: url(\'https://cn.bing.com'+data.images[k].url +'\')">'+data.images[k].title+'</div>')
}
},
error: function () {
$('.d0').text("接口请求失败");
}
})
</script>
</body>
</html>
9.2回调函数
- 回调函数:参数引用一个函数,并将数据作为参数传递给该函数。
- jqXHR:一个XMLHttpRequest对象
参数 | 函数格式 | 描述 |
---|---|---|
beforeSend | function(jqXHR,object) | 发送请求前调用的函数,例如自定义http头 |
success | function(data, String textStatus,jqXHR) | 请求成功后调用的函数,参数data: 可选,由服务端返回的数据(JSON) |
error | function(jqXHR,String textStatus,errorThrown) | 请求失败时调用的函数 |
complete | function(jqXHR,String textStatus) | 请求完成后(无论成功与否)调用的函数 |
Last updated 05 Nov 2024, 01:25 +0800 .